Copia el següent codi i fes els exercicis.
import arcade
SCREEN_WIDTH = 640
SCREEN_HEIGHT = 480
class Ball:
""" This class manages a ball bouncing on the screen. """
def __init__(self, position_x, position_y, change_x, change_y, radius, color):
""" Constructor. """
# Take the parameters of the init function above, and create instance variables out of them.
self.position_x = position_x
self.position_y = position_y
self.change_x = change_x
self.change_y = change_y
self.radius = radius
self.color = color
def draw(self):
""" Draw the balls with the instance variables we have. """
arcade.draw_circle_filled(self.position_x, self.position_y, self.radius, self.color)
def update(self):
""" Code to control the ball's movement. """
# Move the ball
self.position_y += self.change_y
self.position_x += self.change_x
# See if the ball hit the edge of the screen. If so, change direction
if self.position_x < self.radius:
self.change_x *= -1
if self.position_x > SCREEN_WIDTH - self.radius:
self.change_x *= -1
if self.position_y < self.radius:
self.change_y *= -1
if self.position_y > SCREEN_HEIGHT - self.radius:
self.change_y *= -1
class MyGame(arcade.Window):
def __init__(self, width, height, title):
# Call the parent class's init function
super().__init__(width, height, title)
# Set the background color
arcade.set_background_color(arcade.color.ASH_GREY)
# Attributes to store where our ball is
self.ball = Ball(50, 50, 3, 3, 20, arcade.color.AUBURN)
def on_draw(self):
""" Called whenever we need to draw the window. """
arcade.start_render()
self.ball.draw()
def update(self, delta_time):
""" Called to update our objects. Happens approximately 60 times per second."""
self.ball.update()
def main():
window = MyGame(640, 480, "Drawing Example")
arcade.run()
main()
Exercicis
- Crear una altra bolla, d’un altre color.
- es crea a init amb self.ball2(paràmetres)
- Fer que la bolla es mogui, al codi de MyGame a l’objecte self.ball2
- fer update
- fer draw
- Crea l’objecte arbre dels exercicis anteriors, fes que surti a la pantalla.
- Canvia la velocitat de la bolla nova.
- Crear un nou mètode a ball per canviar de color.
- Crear una funció de canvi de color aleatori.
- S’ha de posar un “colisionador” que detecti quan una bolla toca a una altra.
- Cream una classe nova que ens calcularà les colisions.
Solució colisionador.
La funció de colisió ha de retornar si colisionen dos objectes amb un true i un false. Ho calculam mitjançant la fòrmula de distància. La fòrmula:
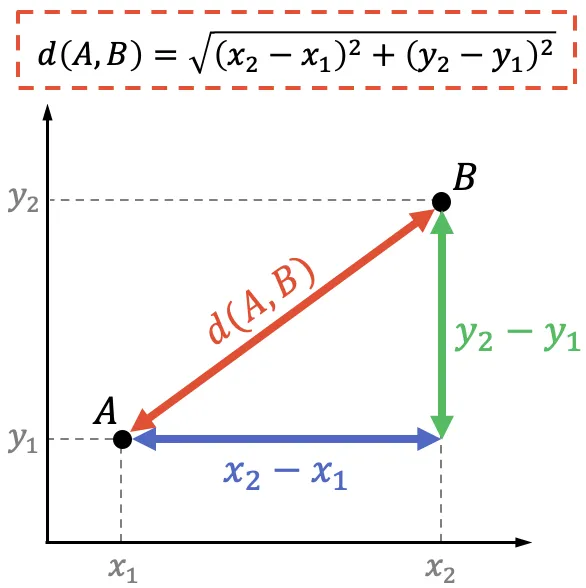
- ELS PUNTS A I B SERAN LES BOLLES DEL NOSTRE PROGRAMA, (CENTRE + RADI)
- PER OBTENIR AQUESTES DADES HO MIRAM DINS LA CLASSE BOLLA:
- def init(self, position_x, position_y, change_x, change_y, radius, color)
- Cada objecte Bolla
- 7.1 Cream la funció colisionador. La funció retrona true quan els objectes colisionen. Aquesta té un petit error de distàncies, a veure si la trobes:
def colisio(self,a,b):
distancia = math.sqrt(math.pow((a.position_x - b.position_x),2) + math.pow((a.position_y - b.position_y),2))
#print(distancia)
if distancia <= (a.radius):
return True
else:
return False
- 7.2 Per controlar la colisió em de